10 Best Practices for Developing Professional Django Projects
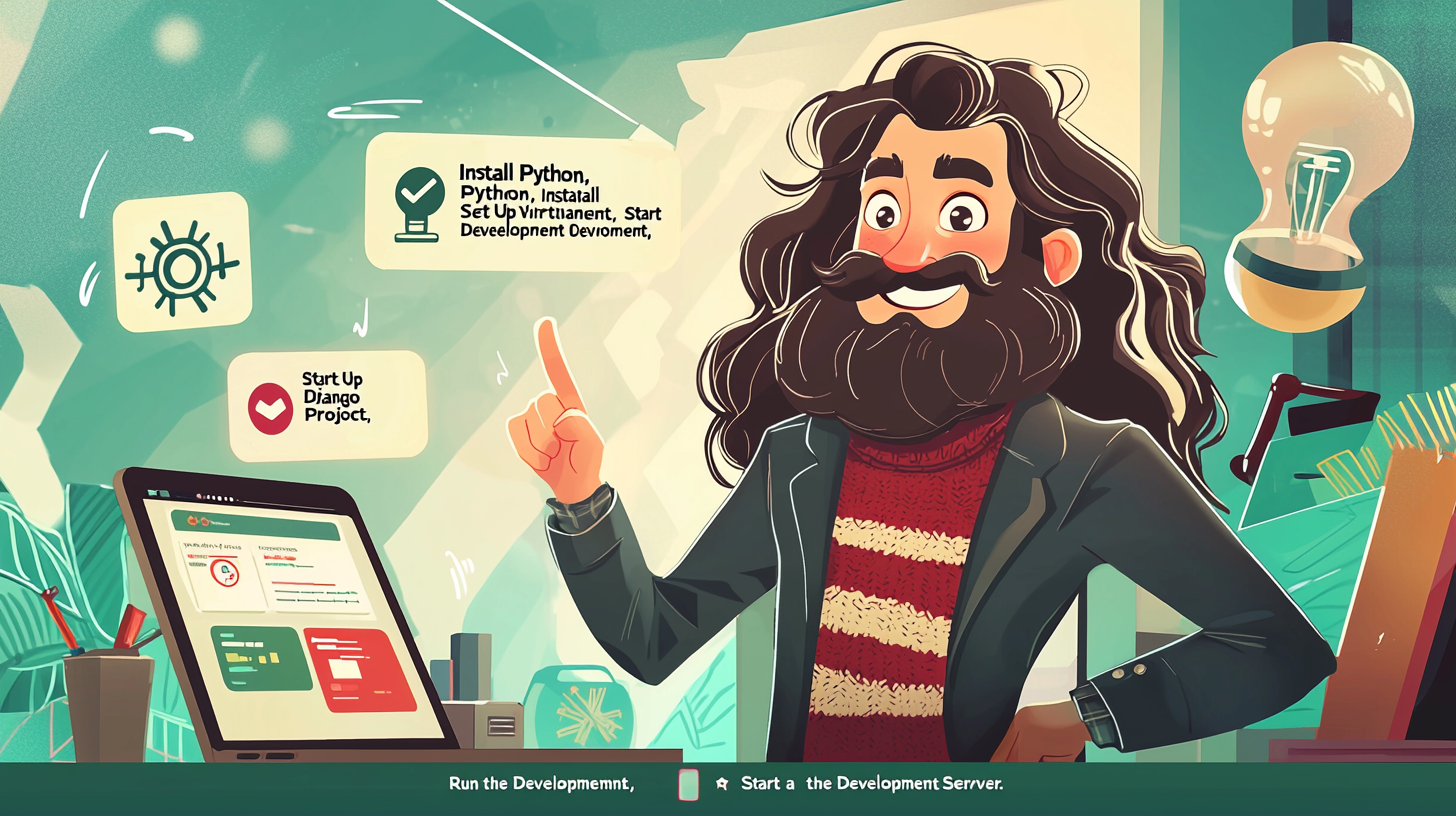
If you’re diving into the world of Django development, you’re in for a treat. 🎉 This guide will help you write clean, efficient, and maintainable Django projects with tips that professional developers swear by.
Whether you’re building your first app or managing a large-scale project, following these best practices will make your life easier and your code better. Ready to level up? Let’s go! 🚀
"Any fool can write code that a computer can understand. Good programmers write code that humans can understand." - Martin Fowler
The 10 Essential Best Practices
1. Use Virtual Environments
Picture this: You’re working on Project A, but its libraries conflict with Project B. Disaster, right? That’s why virtual environments exist.
Virtual environments isolate dependencies for each project, ensuring you avoid conflicts. Think of them as dedicated toolboxes for every project.
# Create and activate a virtual environment
python -m venv venv
source venv/bin/activate # On Windows: venv\Scripts\activate
2. Use Environment Variables
Hardcoding secrets like API keys and database passwords is a rookie mistake (and a security nightmare). Instead, store sensitive information in a .env file.
Tools like python-dotenv let you load variables seamlessly:
# Load environment variables
from dotenv import load_dotenv
import os
load_dotenv()
SECRET_KEY = os.getenv("SECRET_KEY")
Bonus tip: Add .env to your .gitignore to keep it out of your repo.
3. Follow the DRY Principle
DRY stands for Don’t Repeat Yourself. Repetition in code makes it harder to maintain and debug. Extract reusable logic into functions or mixins.
Trust me, your future self will thank you when you’re not rewriting the same logic five times.
4. Organize Your Apps Properly
Keep your project modular by dividing features into apps. For example:
- users/ - Authentication and user management.
- blog/ - Blog features and posts.
- orders/ - Handle order processing and payments.
A clean structure makes debugging and scaling far more manageable.
5. Optimize ORM Queries
Django’s ORM is powerful, but misuse can slow your app down. Avoid the dreaded N+1 query problem with tools like select_related and prefetch_related.
# Optimized query to fetch related objects
Product.objects.select_related('category').all()
Faster queries = happier users. 🚀
Conclusion
Developing professional Django projects isn’t just about writing code; it’s about writing great code. By following these 10 best practices—like using virtual environments, optimizing queries, and organizing your apps—you’ll save time, reduce headaches, and impress your team.
What’s next? Pick one practice, apply it to your project today, and watch the magic happen. Let me know in the comments which one you’re trying first!
Need Help With Your Django Project?
Whether you're starting a new app, fixing bugs, or optimizing performance, I’ve got your back. Let’s work together to turn your ideas into solid, scalable solutions.
Share this article

Erik Taveras
Backend Developer
Helping businesses build secure, scalable backend systems. Specialized in Python, Django, and PostgreSQL.
Work with meRelated Articles
No related articles found.
Stay Updated
Get notified about new articles and resources.
I respect your privacy. Unsubscribe at any time.
Need Help With Your Project?
I specialize in building secure, scalable backend solutions for businesses.
Get in Touch