Building Your First Django Project: A Step-by-Step Guide
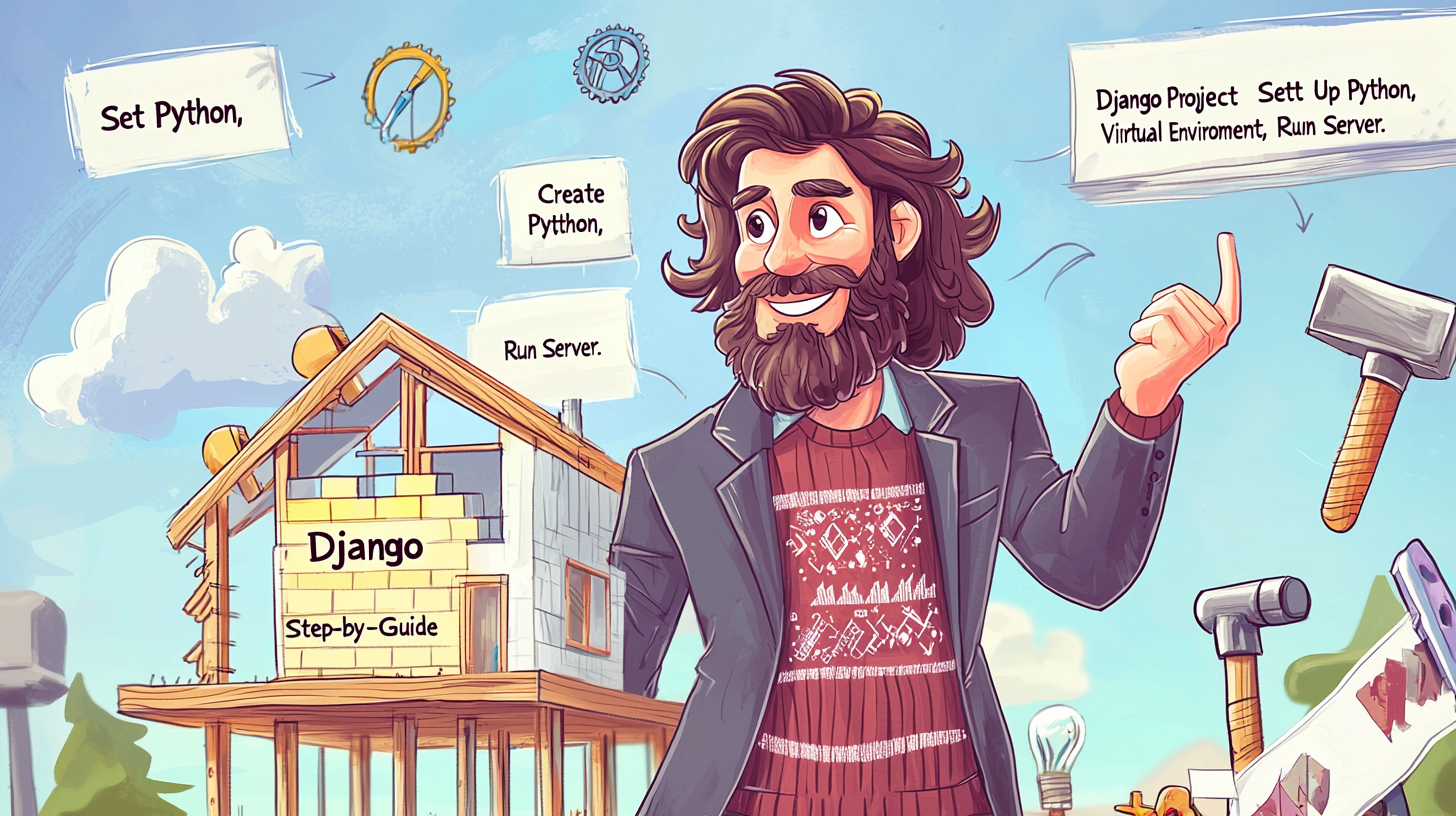
Welcome to this step-by-step guide to building your first Django project! 🚀 Django is a powerful web framework that simplifies the development of robust web applications. Whether you're a beginner or someone revisiting the basics, this guide will take you from setup to a fully functioning Django application.
By the end of this tutorial, you’ll have a clear understanding of Django’s core components, including project structure, views, templates, and URLs. Ready to build something amazing? Let’s dive in!
"The only way to learn a new programming language is by writing programs in it." - Dennis Ritchie
Step 1: Setting Up Your Environment
Before starting your project, you need to prepare your environment. Django relies on Python, so ensuring you have the right tools installed is critical. Follow these steps:
- 💻 Install Python 3.10+ from python.org.
- 📦 Ensure pip is installed (it comes with Python).
- 🔧 Use
venv
orvirtualenv
to create isolated environments for your projects.
Once you have Python installed, run the following commands to set up your project folder and virtual environment:
# Create a new directory and navigate into it
mkdir my_django_blog
cd my_django_blog
# Set up and activate a virtual environment
python -m venv env
source env/bin/activate # For Windows: env\Scripts\activate
# Install Django
pip install django
Congratulations! Your environment is ready. Let’s move on to creating your first project. 🎉
Step 2: Creating Your Django Project
A Django project is a collection of settings and configurations for an instance of the Django framework. It’s the foundation of your application.
Create a new Django project by running:
# Create a new Django project in the current directory
django-admin startproject myblog .
This command generates the following structure:
myblog/
manage.py
myblog/
__init__.py
settings.py
urls.py
asgi.py
wsgi.py
manage.py
: A command-line utility for managing your project.settings.py
: Contains your project’s configuration settings.urls.py
: Handles URL routing for your application.
Run your server to test the setup:
python manage.py runserver
Open http://127.0.0.1:8000 in your browser to see the default Django welcome page. 🎉
Step 3: Creating a Django App
A Django app is a modular component of your project. Each app handles a specific function, making it easier to scale and manage your project.
Create your first app by running:
# Create a blog app
python manage.py startapp blog
Add the app to your project by registering it in settings.py
:
# settings.py
INSTALLED_APPS = [
...
'blog',
]
Step 4: Creating Views and URLs
A view in Django is a Python function that takes a request and returns a response. URLs link requests to views.
Add a simple view in blog/views.py
:
# blog/views.py
from django.http import HttpResponse
def home(request):
return HttpResponse("Welcome to My Blog!
")
Create a urls.py
file in the blog
app and define a URL pattern:
# blog/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='home'),
]
Link the app's URLs to the project by including it in myblog/urls.py
:
# myblog/urls.py
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('blog.urls')),
]
What’s Next?
Congratulations! 🎉 You've successfully built the foundation of your first Django project. From setting up your environment to creating views and URLs, you've taken significant steps into the world of web development. Each line of code you've written today is a building block for more complex and exciting projects in the future.
In the next part of this series, we’ll explore Django templates, delve into models for managing your database, and even learn how to style your app with CSS. Stay tuned as we continue to build on this foundation and bring your ideas to life.
"Every great developer you know got there by solving problems they were unqualified to solve until they actually did it." - Patrick McKenzie
Remember, every project starts small, but with consistent effort, you'll master the art of building powerful and scalable web applications. If you have questions, need guidance, or simply want to share your progress, don’t hesitate to reach out.
💬 Prefer chatting? Feel free to connect with me on social media or drop a message via the contact page. Let’s build something amazing together!
Share this article

Erik Taveras
Backend Developer
Helping businesses build secure, scalable backend systems. Specialized in Python, Django, and PostgreSQL.
Work with meRelated Articles
No related articles found.
Stay Updated
Get notified about new articles and resources.
I respect your privacy. Unsubscribe at any time.
Need Help With Your Project?
I specialize in building secure, scalable backend solutions for businesses.
Get in Touch